A java implementation of Little AI game
Resources :
source files
Eclipse workspace
executable JAR
Description :
This version was developed in Java, with the aim of allowing easy creation of levels based on complex environments.
The game interface is always organized in the same way: the button panel allowing to interact with the environment is on the bottom left. The environment is on the right, in a black box: the player can only explore it through available interactions. At each enacted interaction, the player can observe the result, under the form of an interaction, with the associated score. The global score is displayed at the top left, and display the sum of the last ten interactions. The goal of the player is to understand the rules of the environment by analyzing sequences of interactions, in order to maximize the score.
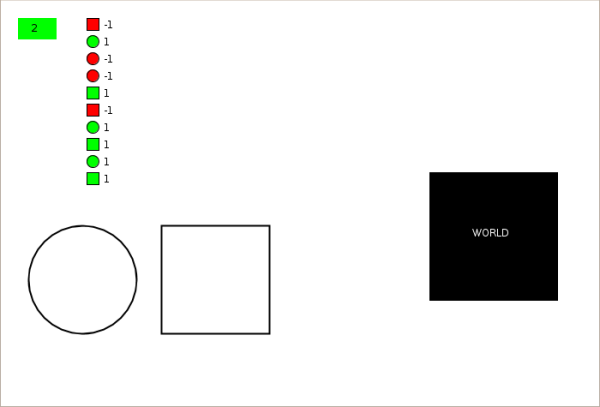
If you reach a sufficient score, you will be allowed to observe the environment. Does it correspond to your assumptions?
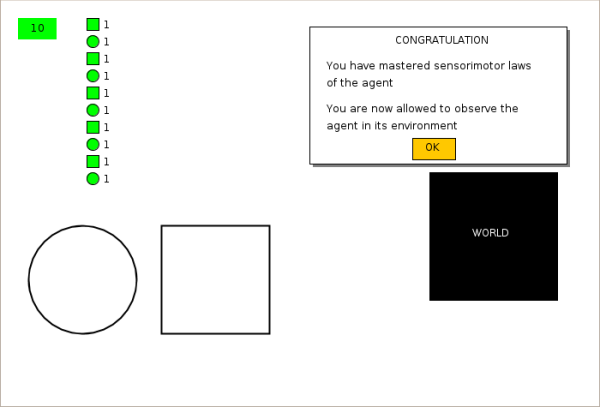
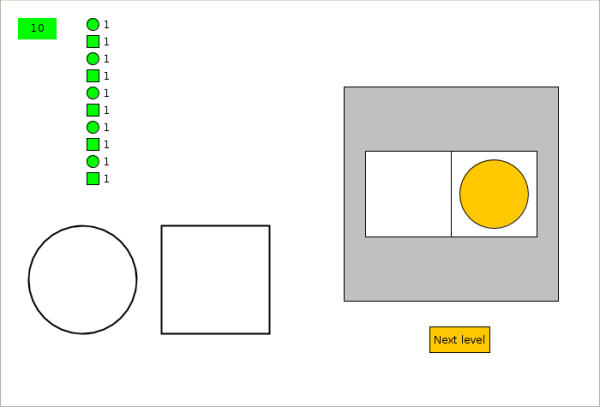
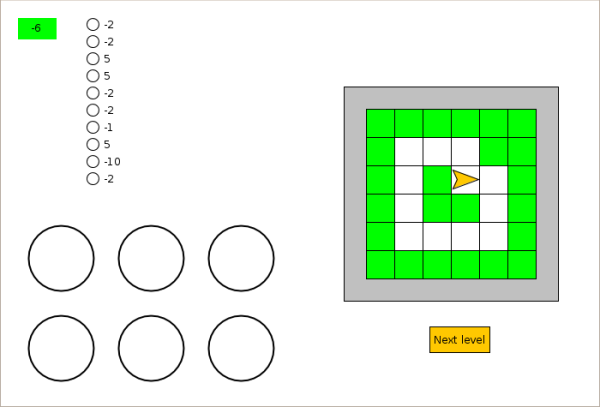
How to create a level?
The first step consists in defining the environment with which the avatar of the player will interact. The environment is composed of a matrix of numbers. Then, you need to define the possible actions, and their results according to values in cells of the matrix. Each interaction is defined with a number, from 0 to n, and each action with a number from 0 to m.
Once the environments and its properties are defined, you can implement it. To create a level, you need to create a new class, inheriting class Level (as an example,
Level3
). This is the minimal frame to create a new level:
import java.awt.Color;
public class Level3 extends Level{
public Level3(){
//initialize variables
}
public void action(int a){
int[] ret=new int[1];
// description of interactions
push(ret);
}
public boolean solved(){
// conditions of success
return sum>=10; // example : when score reaches 10
}
public void drawAgent(Graphics2D g, float x, float y, int width){
// draw the avatar
}
}
Initialization of variables : several variables must be defined to describe and initialize the environment:-
int nbActions
: defines the number of available actions-
int nbInteraction
: defines the number of possible interactions-
int[] actionMap
: vector of length nbInteraction giving, for each interaction, the associated action-
int[] valence
: vector of length nbInteraction giving the score associated to each interaction-
int panel_width
and int panel_height
: gives the number of columns and lines of the button panel-
int[] shapes
: vector of length nbAction giving the initial form of each action. default: 0->circle, 1->square, 2->triangle top, 3->triangle bottom, 4->triangle left, 5->triangle right-
int[] colors
: vector of length nbInteraction giving the initial color of each interaction. Default: 0->white, 1->black...-
int[][] world
: matrix defining the environment-
int px
, int py
, float theta
: initial position (x,y) and orientation of the avatar in the environment.-
Color color
: color of the avatar, when displayed. By default, the avatar is orange.method
public void action(int a)
: this method defines the "physical" properties of the environment, and describes the avatar-environment interaction that happens when the player selects action a
. As an example, it is possible to move the avatar, or change the value of a cell of the environment.
Vector int[] ret
is the vector containing the id of the interaction(s) considered as enacted. Note that this vector must have a constant size. The last instruction, push(ret)
, records the enacted interaction(s).function
public boolean solved()
: defines the success condition of the level. Two variables can be used: sum
, giving the score, and tests
, counting the number of actions of the player. It is also possible to define the success as the fact of obtaining a given interaction a certain number of times, or to successfully enact a certain sequence of interaction: there are no limits, except your imagination.method
public void drawAgent(Graphics2D g, float x, float y, int width)
(optional) : draws the avatar, at coordinates (x,y) of the matrix, with a size of width. By default, the avatar is represented with a circle.Finally, the last step: integrating the level to the game, by adding or inserting, in method
void newLevel()
, an additional line: else if (id_level==4) level=new Level3();